I’ve been doing a lot of work on forms currently, hence the multiple form UI posts!
There are many ways in which you can use css to create table-less forms.
The implementation below is a very effective one, that uses <dd> tags, that are more commonly used now that the earlier days of HTML. This tag was standardized in HTML 2.0 and has been existing for a really long time.
To see an example of the usage of the dd tag, refer to http://www.w3schools.com/TAGS/tag_dd.asp
It essentially stands for “Description of items in a definition list”
Here’s what our form will look like after we’re done coding it.
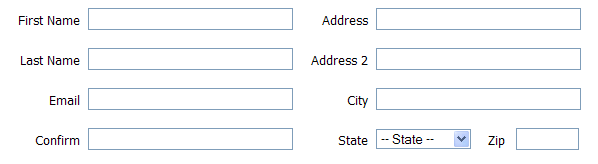
I have tested this on IE6, FF, Safari and Chrome. I should ideally be testing it on IE7 as well, but unfortunately my computer that has IE7 installed has been attacked. Will update the post once I’ve tested it out on that too..
1. Here’s the CSS that needs to go either in a seperate stylesheet or in the head tag of your html file in the <style> tag
/*******************************************
GENERAL CSS FOR FONT SIZE / COLOR
*******************************************/
body, dl, div, p {color:#000; font-family:tahoma, arial; font-size:10pt;}
/*******************************************
FORM CSS – THESE ARE GENERAL DL / DD STYLES
*******************************************/
dl {float:left; position:relative; width:700px; padding:0px; margin:0px;}
dl div {float:left;}
dt {float:left; width:75px; padding:4px 0 2px 0; text-align:left;}
dd {float: left; width:205px; margin: 0 0 8px 0; padding: 0 0 0px 8px;}
dd p {padding:0px; margin:0px; height:10px;}
dt label {width:75px; float:left; text-align:right; display:block;}
input {width:205px;}
/*******************************************************************************************
FORM CSS – THESE ARE SPECIFIC TO CERTAIN FIELDS. IN THIS CASE, TO ACHIEVE THE STATE / ZIP ON
THE FORM. THIS WILL ALSO GIVE YOU A GENERAL IDEA OF HOW TO ADD FLEXIBILITY TO CHANGE
THESE AROUND FOR CERTAIN ELEMENTS ONLY.
*******************************************************************************************/
#Zip {width:118px;}
#Zip dt {float:left; width:44px; padding:4px 0px 2px 0;}
#Zip dd {float: right; width:70px; padding:0px; text-align:right;}
#Zip dt label {width:44px; float:left; text-align:right; display:block;}
#zipcode {width:63px;}
#State dt {float:left; width:75px; padding:4px 0 2px 0; text-align:left;}
#State dd {float:left; width:85px; padding:0 0 0px 8px;}
select {width:95px; height:20px;}
2. Here’s the markup that goes in the <body> of your file.
<dl>
<div id=”FirstName”>
<dt><label for=”firstname”>First Name</label></dt>
<dd>
<input type=”text” id=”firstname”>
<p><!–the backend error can be served here–></p>
</dd>
</div>
<div id=”Address”>
<dt><label for=”address”>Address</label></dt>
<dd>
<input type=”text” id=”address”>
<p><!–the backend error can be served here–></p>
</dd>
</div>
<div id=”LastName”>
<dt><label for=”lastname”>Last Name</label></dt>
<dd>
<input type=”text” id=”lastname”>
<p><!–the backend error can be served here–></p>
</dd>
</div>
<div id=”Address2″>
<dt><label for=”address2″>Address 2</label></dt>
<dd>
<input type=”text” id=”address2″>
<p><!–the backend error can be served here–></p>
</dd>
</div>
<div id=”Email”>
<dt><label for=”email”>Email</label></dt>
<dd>
<input type=”text” id=”email”>
<p><!–the backend error can be served here–></p>
</dd>
</div>
<div id=”City”>
<dt><label for=”city”>City</label></dt>
<dd>
<input type=”text” id=”city”>
<p><!–the backend error can be served here–></p>
</dd>
</div>
<div id=”Confirm”>
<dt><label for=”confirm”>Confirm</label></dt>
<dd>
<input type=”text” id=”confirm”>
<p><!–the backend error can be served here–></p>
</dd>
</div>
<div id=”State”>
<dt><label for=”state”>State</label></dt>
<dd>
<select id=”state”>
<option value=””>– State –</option>
</select>
<p><!–the backend error can be served here–></p>
</dd>
</div>
<div id=”Zip”>
<dt><label for=”zipcode”>Zip</label></dt>
<dd>
<input type=”text” id=”zipcode” class=”textInput”>
<p><!–the backend error can be served here–></p>
</dd>
</div>
</dl>
3. Here’s a very short explanation. The code itself should explain the rest!
-> All the dt / dd tags have been put in their own unique containers. This is done so that you have the flexibility to change the widths / heights of any of the element within that container. For e.g. I’ve applied different width’s / padding / floats to the zip and state fields.
I actually used the above markup to a form that was generated in a loop, giving a unique id to each div. It really helped me position around the elements the way I wanted to without changing the markup. A simple CSS file did the trick!
-> It is very useful to have <label> tags in a form. They help in many ways.
If you want to read more on label tags, see the browser support for it etc, you can refer to http://www.w3.org/TR/html401/interact/forms.html#h-17.9 and / or http://www.w3schools.com/tags/tag_label.asp
4. Download the html / css for this file here – http://www.pluthra.com/blogdata/final-form.html